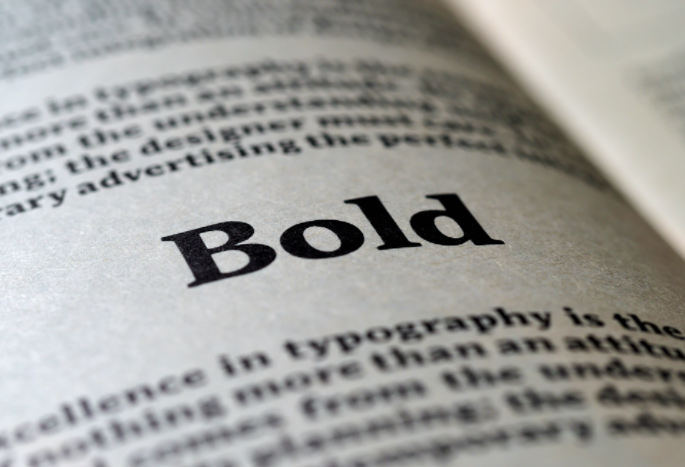
TypeScript’s Record Type Explained
Everything you want to know about the Record utility type
Record is one of the TypeScript utility types and has been available out of the box since version 2.1.
I have to admit that I was a little confused when I read the official definition for the first time:
“Record<Keys,Type>
Constructs an object type whose property keys are Keys and whose property values are Type. This utility can be used to map the properties of a type to another type.” — TypeScript’s documentation
At face value, it says the Record type creates an object type that has properties of type Keys with corresponding values of type Type. But a similar feature can be achieved by using an index signature, so why would we want to use a Record type? What makes it different or useful?
Record Type vs. Index Signature
In TypeScript, we call the way of accessing an object property with square brackets an index signature. It is widely used for object types with unknown string keys and a specific value type. Here is an example:
type studentScore= { [name: string]: number };
The index signature example above can be expressed by a Record type as well:
type studentScore = Record<string, number>;
For this use case, the two type declarations are equivalent from a type-asserting perspective. But from the syntax perspective, the index signature is better. In the index signature approach, the name key expresses the intent more clearly, and another advantage is that it is shown in VisualCode IntelliSense.
Then why do we want to use a Record type?
Why is the Record type useful?
The benefit of Record types are conciseness. It is particularly useful when we want to limit the properties of an object. For example, we can use a union of string literals to specify the allowable keys for the Record type, as shown below:
In this example, we define a type with a union type constraint. If we try to access a property that is not in the roles union type, the VS Code compiler won’t be happy. The compile time check is very useful when we maintain a complex type, as the compiler will prevent these sorts of mistakes from happening.
Another useful feature is that the keys can be enums as well. In the following example, we use the staffTypes enum as a restricted key of the Record type, so it is more readable. Please note that enums are only supported after TypeScript 2.9. As such, the type of key is restricted to string type before version 2.9.
Combining the Record Type With keyof Operator
keyof operator is a type operator, which can extract the object keys as a union type. By using keyof** **to get all properties from an existing type and combine it with a string value, we could do something like the following:
It is handy when you want to keep the properties of an existing type but transform the value type to others.
Advanced Usage Example
A Record type can be used together with other utility types for more advanced use cases. Here is an example:
1 | type seniorRole = 'manager'; |
With Record, Partial, and Intersection types working together, this code creates a strongly typed benefits object with an association between keys and value types. The strongly typed object makes it possible to catch the error at compile time. It also makes it possible for the IDE to flag errors while typing and provides IntelliSense with auto-completion.
Summary
Record is a handy and concise utility type that can make your code more robust. It is especially useful when dealing with a dynamic data structure. The goal of Record type is the same as the other wonderful TypeScript features: better type safety .
If you like this article, you may also like to read another article about infer keyword .
If you are not already a paid member of Medium, **you can do so by visiting this link **. You’ll get unlimited full access to every story on Medium. I’ll receive a portion of your membership fees as a referral.